Building a Simple Q&A App with Gemini Pro and Python
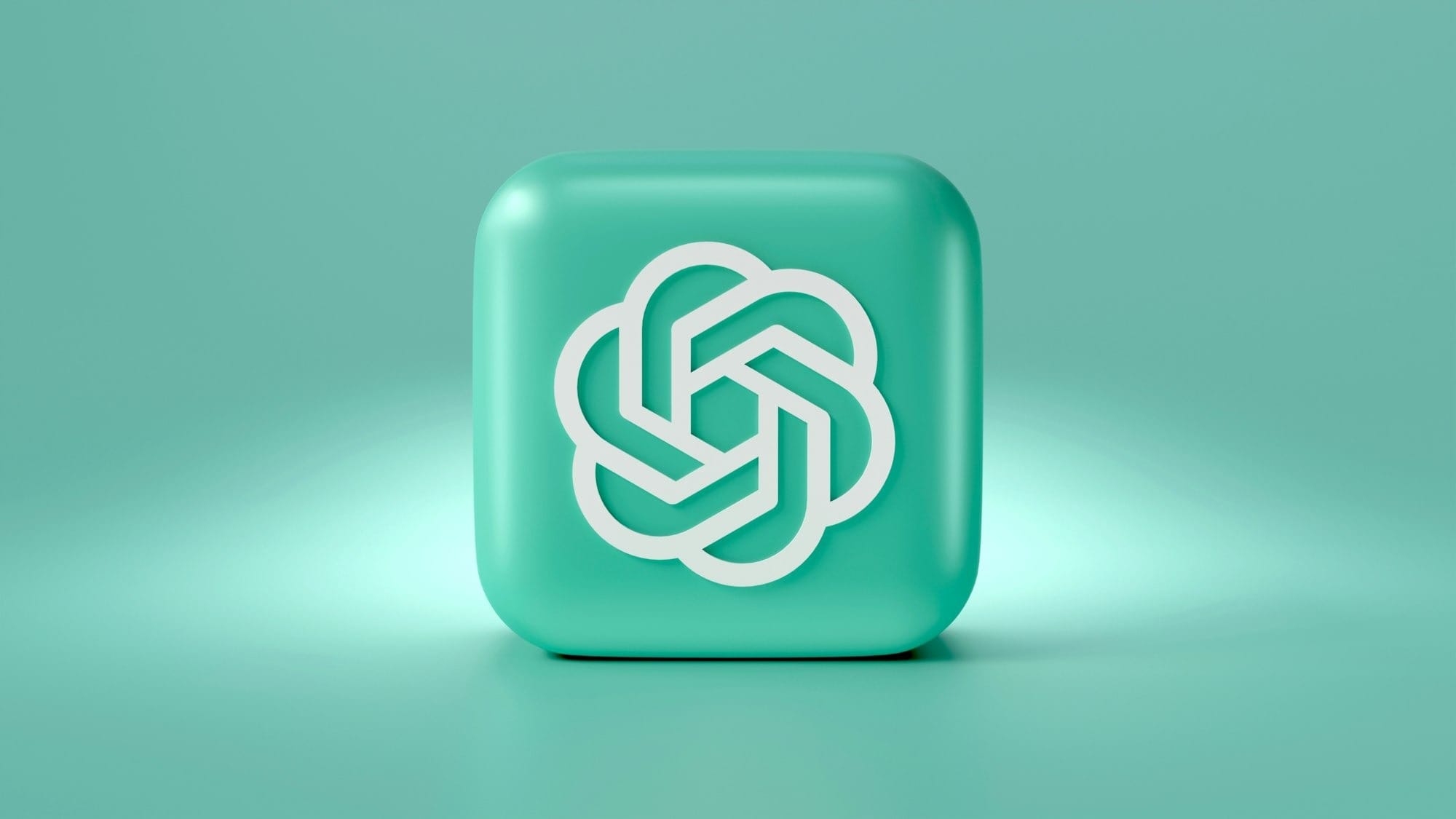
Step 1
Before everything, you need to have API key fto use Gemini models. If you dont know how to create one, reffer this blog post.
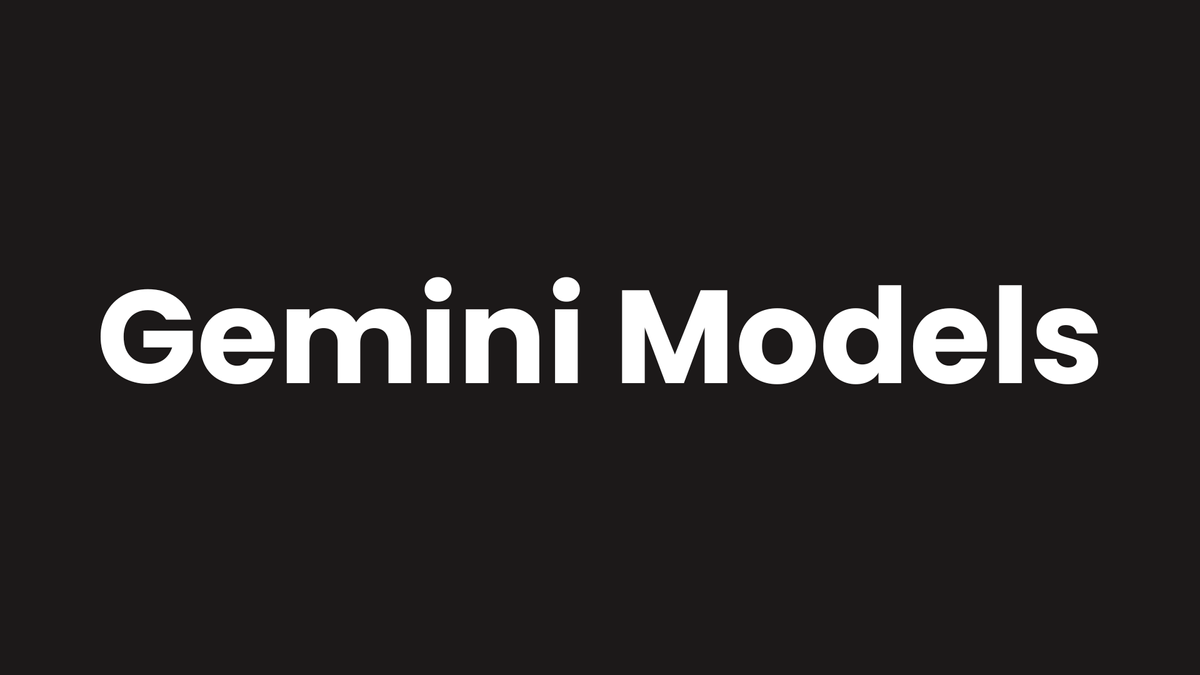
Step 2
Create a virtual environment Using a virtual environment helps keep your project dependencies separate. Here are two ways to do it:
- With conda:
conda create --name gemini-env python=3.9 # Create environment
conda activate gemini-env # Activate the environment
- With Python:
python -m venv gemini-env # Create environment
source gemini-env/bin/activate # Activate the environment
# On Windows, use: gemini-env\Scripts\activate
Step 3
Create a file named 'requirements.txt' with these packages
streamlit
google-generativeai
python-dotenv
Why use these packages?
- Streamlit - Creates web apps easily with Python. It's great for quick prototypes and data apps.
- google-generativeai - Official library for using Google's AI models, including Gemini Pro.
- python-dotenv - Loads environment variables from a file. This keeps sensitive info (like API keys) separate from your code.
Step 4
Run this command to install the required packages
pip install -r requirements.txt
Step 5
Make a file named .env
and add your API key
GOOGLE_API_KEY="YOUR_API_KEY_HERE"
This keeps your API key secure and separate from your code.
Step 6
Create a new Python file (e.g., 'app.py') and add these imports
from dotenv import load_dotenv
load_dotenv()
import streamlit as st
import os
import google.generativeai as genai
Here's what each import does:
- dotenv - Loads environment variables from .env file
- streamlit - Creates the web interface
- os - Accesses environment variables
- google.generativeai - Interacts with the Gemini models
Step 7
Choose your model.To see available models:
for x in genai.list_models():
print(x.name)
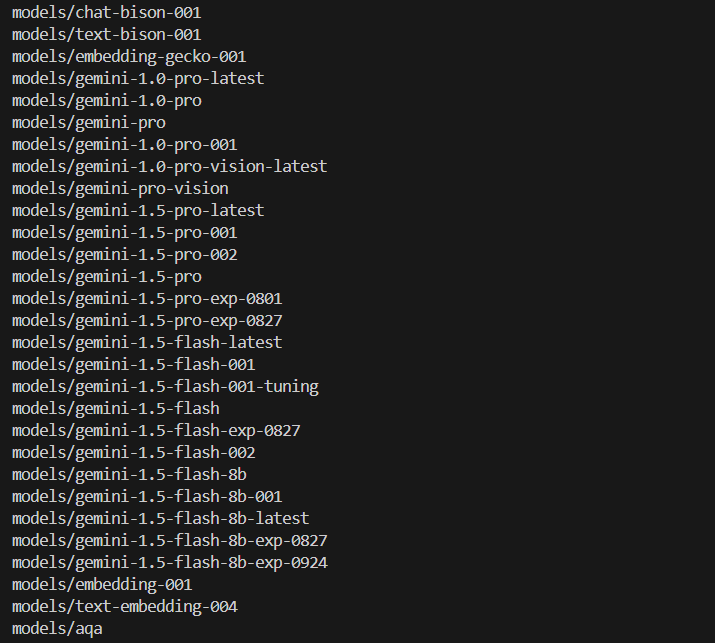
We'll use gemini-pro
for this project.
Step 8
Configure API key Set up the API key for use
genai.configure(api_key=os.getenv("GOOGLE_API_KEY"))
This line gets the API key from the environment variable and configures the genai library.
Step 9
Load the model Initialize the Gemini Pro model:
model = genai.GenerativeModel('gemini-pro')
This creates an instance of the Gemini Pro model for generating responses.
Step 10
Create a function to get responses Define a function to interact with the model:
def get_gemini_response(question):
response = model.generate_content(question)
return response.text
This function takes a question, sends it to the model, and returns the generated response.
Step 11
Set up the Streamlit app Create the user interface
st.set_page_config(page_title='MYGPT')
st.header("Simple Gemini Application")
input = st.text_input("Write me something: ", key='input')
submit = st.button("Get Answer")
This code:
- Sets the page title
- Adds a header
- Creates a text input field
- Adds a submit button
Step 12
Handle response and display Process the user's input and show the result:
if submit:
with st.spinner('Generating response...'):
response = get_gemini_response(input)
st.write(response)
This code
- Checks if the submit button is clicked
- Shows a loading spinner while generating the response
- Displays the model's response
That's it! You now have a simple Q&A application using the Gemini Pro model. To run it, use the command.
streamlit run app.py
This will start a local web server and open the app in your browser. You can type questions or prompts, and the Gemini Pro model will generate responses.
Watch this video for a demo
Remember to keep your API key secret and never share it publicly.
Happy coding!